In the previous blog entry we successfully got data returned to our view control. At this point we have gone from a blank module to having a functioning web service created within a module which we then consumed in our view.ascx file. We’ve taken several steps, created a database, classes, controllers, and web services. Things may have moved a little quick or perhaps a little slow for you or it may have been exactly the right pace. In case some of these previous materials we reviewed are a little fuzzy, don’t worry too much about it because you will continue to learn the more and more you get into it. I’m proof of that (and I’m still learning as you see in the videos)!
This blog entry is going to be a non-code blog entry where we just review how things work thus far. We are reviewing because your gaining understanding of concepts and learning is my ultimate goal in this series. So let’s review and talk about the steps we’ve taken thus far.
The Software
The first thing we did was ensure that we had the appropriate software on our machines in order to be able to develop a DNN module. All of the software we developed on top of, also referred to as “the stack”, was Microsoft technology. Sometimes, when developers first meet each other, they ask the question “Hey what stack do you develop on?” We are definitely developing on the Microsoft stack.
The softwares we installed and/or made sure we had ready were Microsoft Visual Studio, SQL Server, and we also made sure that IIS was properly installed and configured. As you saw while we went through the first couple of blog entries in this series, Visual Studio is where we develop most of the code for our module. SQL Server is where our database, tables, and stored procedures reside and IIS essentially hosts the site locally on our machine by binding a URL to the site and it provides our application with the necessary resources to run.
The Code
Thus far in our module development journey we’ve used HTML, CSS, Javascript, JQuery, C#, T-SQL, & ASP.NET at various locations within our module. In the following list of items I want to call out each file and discuss how they are linked to each other so that we can fully understand how the components of our module work together. Again, mentally mapping all this stuff together was one of the areas that was difficult for me as I initially learned and I hope to add clarity in this area for anyone who may have similar difficulties.
View.ascx:
The view.ascx file is the initial user control that is loaded whenever someone accesses a page on which our module resides. This is where we placed our HTML input elements where our users can enter data. This file is where we also returned to put in some jQuery to retrieve our data from our web service. So the scenario goes like this… a user visits a page that our module is on, the view control gets loaded, and any code we have in the view control gets executed.
Module.css:
The module.css file is the “Cascading Style Sheet” file that styles the elements within our module. We created elements in our view.ascx file that are styled by the css properties in our module.css file. By default DNN looks for a module.css file for any module specific styles.
Edit.ascx:
The edit.ascx file is a file that is usually where any editing of module content would occur. Typically the adding, updating, or deleting of module data happens in this file. Though, since we’ve taken the more client side approach and are aiming for less postbacks we have kind of embedded our adding and editing functionality within our View control. We won’t do too much with this Edit control in this series, but you definitely could if you wanted to. There’s really no right or wrong way (though some people will argue you about that) so how you ultimately structure your module and controls is up to you.
Settings.ascx:
The settings.ascx file is a file that contains any specific settings for our module. We have not yet really done much with this file at this point. Though, we will come back to this and create a setting for our module in just a few more blog entries.
Models Folder
Task.cs:
The Task.cs file is the definition for our task class and from that definition we create task objects. These objects have properties that are defined in the class definition such as TaskName, TaskDescription, isComplete, etc. The Task class represents our task objects in memory and the properties of this class parallel with the task data that exists in our Tasks table in SQL.
TaskController.cs:
The TaskController class defines methods/functions that affect our task objects. We currently have a “GetTasks” function within our TaskController. I drew the parallel that the TaskController class being like a traffic director of sorts between the database and our task objects. The methods in the TaskController are usually related to stored procedures in our SQL database.
Webservices.cs:
The Webservices.cs class file contains the logic for our web services. We set up the level of security required for each web service as well as defined the type of request to which our web services respond. We then create responses that get returned to our web service route.
RouteMapper.cs:
The RouteMapper.cs class file configures the routes for our web services. Since web services respond to unique URLs within our site the RouteMapper.cs class allows us to configure portions of the specific path to which our module’s web services will respond. Using the RouteMapper also helps to ensure that no clashes with other web services running our site occur.
Data Provider Folder
00.01.SQLDataProvider:
This file contains the T-SQL scripts that will run anytime our module is installed, upgraded, or uninstalled. As our module captures data entered by the user, the scripts contained in this file will create the necessary tables and stored procedures to house and interact with that data. Also note that in order to work with DNN the SQL Scripts that we generated in SQL Server had to be updated with some DNN specific syntax. At the end of this series we will package our module and install it into a different DNN environment to illustrate the functionality of this file.
Uninstall.SQLDataProvider:
This file contains the T-SQL scripts that will be executed whenever our module is removed from a DNN installation. If in fact a host user uninstalls our module then we want to delete any tables and stored procedures associated with our module. The scripts contained in this file do just that and remove all data associated with our module.
Mentally Mapping the Relationships
With a high level review of the files and what they mean it may also be beneficial to mentally map out how these files interact in a real world scenario. The scenario would occur like this:
- User lands on the website & clicks to a page where our module is installed
- The code in our View.ascx file is executed
- The JQuery script in our View.ascx file reaches out to a specific URL issuing a “HttpGet” request and expects an array of JSON objects in return.
- The specific URL, our web service route, verifies that it responds to that specific request type and that the requesting party has permission to access the information. When confirmed it then executes a method found in our controller class which then reaches out to SQL Server database and runs a stored procedure (the GetTasks stored procedure). The stored procedure returns the expected data and it is posted to the route as an array of JSON objects
- The JQuery script in the View.ascx then gets the array of JSON objects and parses it out into individual JSON objects. The script then loops through each object and dynamically creates a new DIV within our View.ascx with the information found in the JSON object which, in the end, is our list of Tasks.
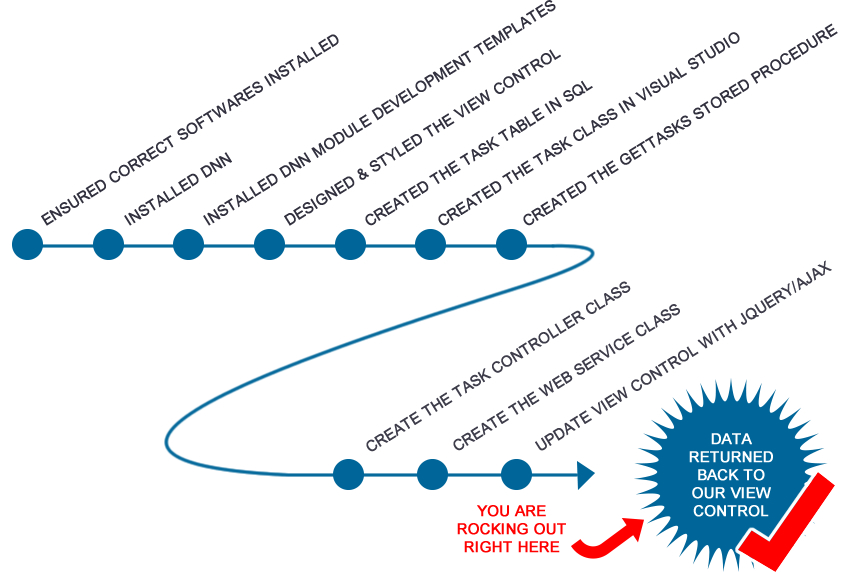
Summary
Hopefully this blog entry has helped you understand how our module functions a bit more clearly. I know in the past I often felt like I had a good grasp on one concept, but then others were just floating around in my mind and I could never tie everything together. This blog is aimed at eliminating that very scenario. For me, I need to know where something lives and what it does in order to mentally be able to move ahead. Until I can get a firm and clear understanding it just drives me crazy. My goal in being so thorough with everything here is to not drive you crazy and eliminate as much grey area and fogginess as I can.
Next up we will learn how to debug our module using Visual Studio’s debugging capabilities. It will be a short blog on debugging and then we’ll get back to it with our quest and make the “Add Task” functionality work for us. See you in the next blog!
Go To The Next Blog Entry: Debugging a DNN Module