In this blog entry we’ll discuss and walk through the process of creating the Task “Controller” class. Thus far we’ve taken several steps and at this point you may be conceptually lost with where we are and what we’re doing. I remember reading tutorials and being able to follow along and click what the instructor/blogger told me to click, but I had no understanding as to what was going on or why I was clicking everything. So let’s take a step back for a second and assess our current status within our module development journey so as to hopefully increase conceptual understanding.
Our Journey Thus Far
After getting the appropriate softwares installed on our machine we installed a DNN module development template which helps us efficiently get started developing modules. We looked at all the components of the module development template, installed the module, and designed and coded the beginnings of our view control. The view control had some placeholder text in it and we need to replace that text with real data.
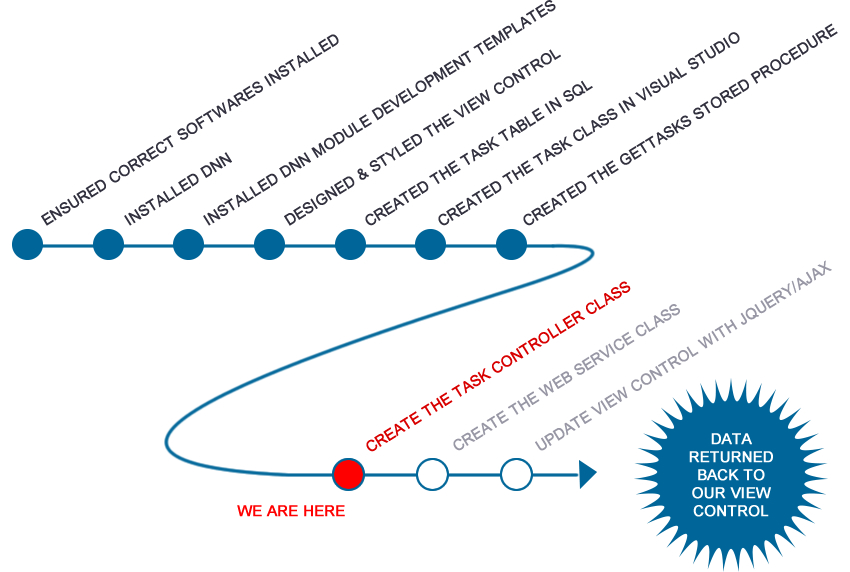
So we started down the path of replacing this “dummy” data with real data which took us to SQL Server. We created a tasks table to hold our task data. Once our table was created with all the appropriate data points we then went back to Visual Studio and updated our SQLDataProvider files so that whenever our module is installed in another DNN site the “Tasks” table our module expects to store data in will be created an available. Then we created the “Task” class which serves as the definition for the objects that will exist in our module’s code. These objects have properties that parallel the same data points we track for our tasks objects and save in our Tasks table in SQL. With our task table and task class both created we then went and created a “GetTasks” stored procedure which handle the repetitive job of retrieving tasks from the Tasks table. The data that is retrieved from this “GetTasks” stored procedure will be returned and that data needs to then populate objects with that data. After creating the stored procedure we, again, went back and updated our SQLDataProvider files this time adding in the necessary scripts to create the “GetTasks” stored procedure whenever our module is installed in a different instance of DNN. This brings us to where we are now with creating the Task Controller class.
The Task Controller Class
The task controller class is the class that controls the objects that the code in our module creates. That is the task controller class will have functions (also called methods) that will take our task objects and send them to the stored procedures when data is being added to the database. The task controller class will also retrieve tasks from the database and then populate objects in memory with the appropriate data.
Analogy: It may be helpful to think of the tasks as being objects that are dynamically created and just floating around in memory and the task controller class as the traffic director of sorts linking or routing the objects to our database table (the Tasks table) and vice versa.
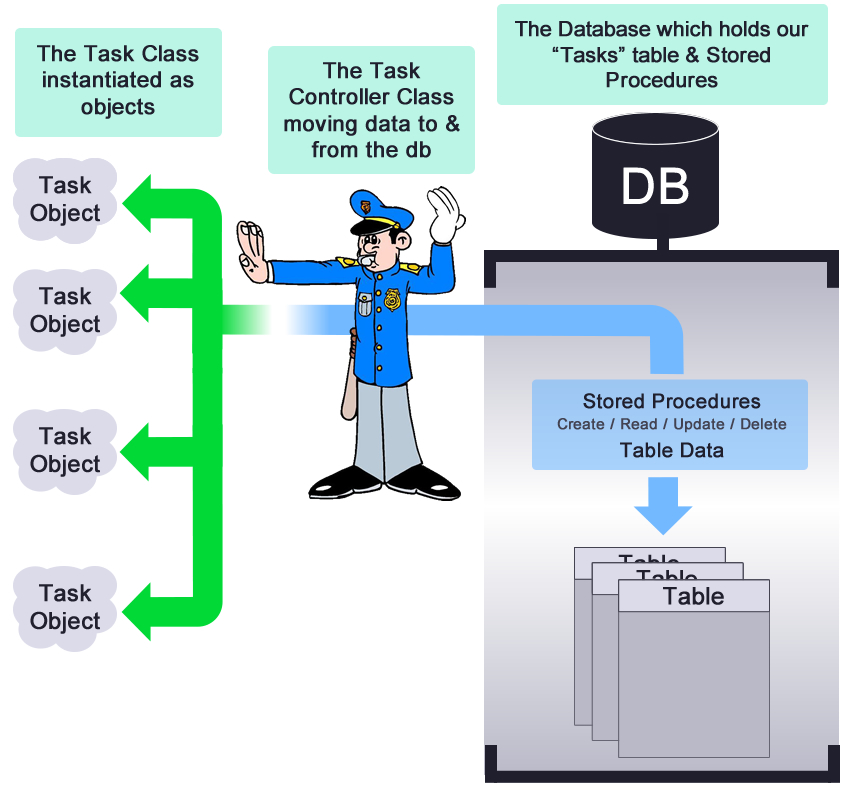
Creating the TaskController Class
To create the TaskController class we’ll do the same as we did before by right-clicking on the “Models” folder and then “Add” then “Class”. Then name the file “TaskController.cs” and click “Add”. Now you’ve got your new TaskController class that resides in the Models folder.
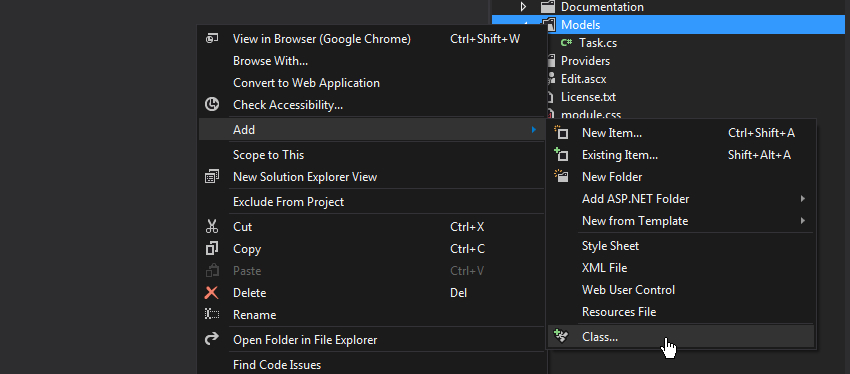
Add New Using Statements
Since we’re going to be dealing with data and some of the DNN API we need to add some additional “using” statements at the top. Currently your class probably has 3 using statements… 1 for System, 1 for System.LINQ, and 1 for System.Web at the very top of the file. Let’s add 3 more to the top.
Add the following:
using System.Collections.Generic;
using DotNetNuke.Common.Utilities;
using DotNetNuke.Data;
We added those using statements because we’re going to be using functionality that those 3 API’s provide. By adding them in before we start coding we’ll get some really helpful intellisense (the code hints or suggestions that Visual Studio provides) that will make coding our module much easier.
Coding the GetTasks Controller
Now we’re ready to code some controllers. #YOLO! If you think about what we’re currently trying to do (which is to return a list of tasks from the database) you can see that we are trying to get multiple tasks… as in more than one task. Since we are trying to get multiple items then we need to create a list of items. So we will create this as an “IList” of type task meaning that it will be a list of task objects. Also keep in mind that we are only interested in returning the tasks for each individual module (remember we are “scoping” our tasks at the module level). Enter the below code snippet into the controller:
public IList<Task> GetTasks(int ModuleID)
{
return CBO.FillCollection<Task>(DataProvider.Instance().ExecuteReader("CBP_GetTasks", ModuleID));
}
Examining that code we see the “Public” “IList” of task objects called “GetTasks” and we are passing in an integer of ModuleID into the list as a parameter. So what does all that mean? The public means that this list can be accessed from outside of this class, IList means we’re creating a list of objects, <Task> represents that this list will be of type Task objects, “GetTasks” is the name of this controller method, and the (int ModuleID) is the parameter that we are accepting because the module ID will vary with each module.
Now in between the squigglies { … } is where the code resides for populating this IList. The “return CBO.FillCollection<Task>” indicates that we will be returning a collection of Custom Business Objects (CBO) of type task. The “(DataProvider.Instance()” portion is using some of the DNN API (remember the DataProvider.cs class file we previously discussed) to ensure that we’re making use of the right data store (our SQL database). The .ExecuteReader is a method that will read data from the database and into that we pass the string of our stored procedure. In this case it’s out “CBP_GetTasks” stored procedure. If you remember the “GetTask” stored procedure expects the module ID as a parameter so we pass that in as well. There you have the connection, in code, from our module to the database (one of the previously very fuzzy areas for me). So when this “GetTasks” controller class function gets called, which we’ll call it in 2 blog entries from now, it will return a list of objects that have their properties populated with the data from our Tasks table in our SQL database. Yes, we are getting close.
Reminder: Just a reminder here that our controller class will eventually have multiple controllers within it. We will need to create controllers to add tasks, update tasks, and delete tasks, but for now we’re simply trying to get data back on the screen.
This video walks through the concepts covered in this blog entry
If you’re having fun and/or have learned something thus far in this series how about tweet to the DNN Community and let us know about it… hey you never know who you’ll meet!
Go to the Next Blog Entry: Module Development vs. "Modern" Module Development