Last week I did a presentation at the DevTeach web developmeent conference in Vancouver. I had hoped that attendees would appreciate the wit in the title of my session, "On the Mobile Web... sometimes RESS is more". Unfortunately, I discovered that the "RESS" acronym is not yet as widely known as I had imagined amongst web developers, so my attempt at being clever was lost on most people. Luckily, the abstract for my session contained a lot more detail and as a result I had a decent turnout for my session.
"With the explosion of mobile devices in recent years (BYOD), organizations of every size are faced with the problem of creating optimized mobile experiences for their online audience... In this session we will cover the various techniques for dealing with the mobile web. We will discuss dedicated mobile websites and the benefits of device detection technology. We will examine responsive web design (RWD) and explore the power of CSS media queries and responsive frameworks such as Bootstrap for customizing the display of content based on the screen size of a device. We will also delve into a new hybrid approach known as Responsive Design + Server Side Components (RESS) which uses a combination of client-side and server-side techniques to provide the best web experience for mobile devices."
I obviously wanted to leverage DNN to showcase all of these techniques in action. And since we have been shipping the 51 Degrees device detection capability in the platform since DNN 6.1, it was very easy to demonstrate how easy it is to detect any mobile device and redirect the visitor to an optimized experience. In my case, I used our multi-site capability to create another site at "m.mysite.com" using the Mobile template, and I created a default redirect rule for mobile devices. And using the new responsive Gravity skin in DNN 7.2 I was able to explain CSS media queries, Bootstrap, and the pros and cons of responsive web design. So that leaves the last technique and the focus of my session: RESS.
RESS stands for Responsive Design + Server Side Components. Essentially what it attempts to solve is the standard responsive design problem where ALL content is sent from the server to the mobile browser regardless of whether that content is expected to be utilized on the client device or not. Sending unnecessary content over the network is extremely inefficient and results in performance issues and a poor user experience. RESS adds an extra layer of intelligence on the server where it detects the device that issued the request and customizes the content that is sent to the client browser.
So how do we enable RESS in DNN?
In DNN 7.0 we introduced a new interface in the core platform named IModuleInjectionFilter. This interface has a single method named CanInjectModule() which provides an extensibility point that is available when a page is being assembled on the server. As DNN iterates through the various modules that are part of a page, it will call the interface for each module instance and allow you to provide custom business logic that will instruct the system on whether or not the module should be injected. This interface can be used for a variety of purposes including licensing, personalization, security, localization, A/B testing, etc... We will use this interface to allow us to specify if a specific module instance should be sent to a mobile device.
Copy the following code and paste it into a new class file named RESSInjectionFilter.cs that you save in the App_Code folder. You will notice that we are utilizing the Header property of each Module intance to provide special instructions on whether the module should be included in the page output or not. We are also taking advantage of the 51 Degrees device detection capability to identify mobile devices on the server.
#region "Using Statements"
using System.Web;
using DotNetNuke.UI.Modules;
using DotNetNuke.Entities.Modules;
using DotNetNuke.Entities.Portals;
using DotNetNuke.Security.Permissions;
#endregion
namespace DotNetNuke.Services
{
public class RESSInjectionFilter : IModuleInjectionFilter
{
public bool CanInjectModule(ModuleInfo objModule, PortalSettings objPortalSettings)
{
bool InjectModule = true;
// RESS instructions stored in module header as an HTML comment <!-- MOBILE=HIDE -->
if (objModule.Header.ToUpper().IndexOf("MOBILE=HIDE") != -1) {
// if user does not have EDIT permissions
if (ModulePermissionController.HasModulePermission(objModule.ModulePermissions, "EDIT") == false) {
// check device characteristics
dynamic objDevice = DotNetNuke.Services.ClientCapability.ClientCapabilityProvider.CurrentClientCapability;
InjectModule = !objDevice.IsMobile;
}
}
return InjectModule;
}
}
}
To enable RESS in our site, we will use a default installation of DNN 7.2 and we will customize the home page so that the large banner images in the rotator are not sent to a mobile device. To do this, we will login to the site and go into Edit mode for the Home page. We will load the Module Settings dialog for the module instance containing the rotating banner. In the Advanced Settings we will add an HTML comment to the Header property of <!-- MOBILE=HIDE -->.
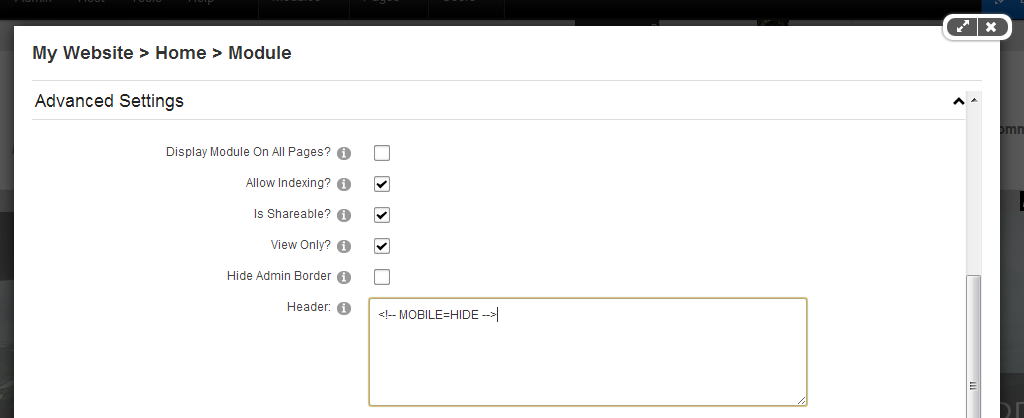
And as a result when we visit the site using a mobile device, the rotating banner will no longer be displayed. This may not seem very impressive on the surface, as it is fairly simply to simply hide content using client-side script. However, the real benefit of RESS is that the content was actually filtered on the server and was never sent across the wire. This reduces the network payload and improves the browsing experiencee for mobile devices significantly. In our example, it reduced the amount of information transferred by almost 500 KB!
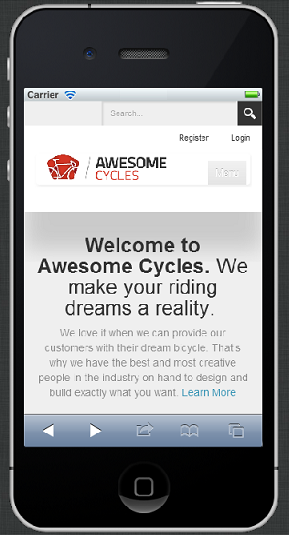
I expect that in the coming year, RESS will get more mainstream adoption and attention as organizations make efforts to optimize their mobile user experience. And on a side note, I hope that this example also showcases the power of the new IModuleInjectionFilter interface which provides extensibility opportunities that were not previously possible in DNN.