First, here is a wonderful article that explains why the Object Data Source control is so great:
http://msdn.microsoft.com/library/default.asp?url=/library/en-us/dnvs05/html/asp2objectdatasource.asp
I am working in a tutorial that will show how to use the new Generic methods in the DAL (we were calling it the “DAL II” but that got confusing with the original DAL II proposal that is not being implemented). Let’s call it the DAL+. The new Generic methods are planned to be in the next DotNetNuke release and will be in both the DotNetNuke 3 and DotNetNuke 4 versions.
Anyway, I first have to make a module then create a tutorial. I didn’t want to use the “Guestbook” example because I have used it for the past 2 years and even I am getting sick of it :). Also, I have learned that a tutorial should be as small and as short as possible. I wanted this tutorial to only have one web control.
The idea I came up with was “ThingsForSale”. This is a simple module with only one control. It allows a person to post a message indicating they have something to sell. They can only edit and delete their own posts (presumably they will delete the post when the item has been sold). The web Administrator can edit and delete all posts. The Administrator will do this from the main screen, no need to go to a separate administration screen.
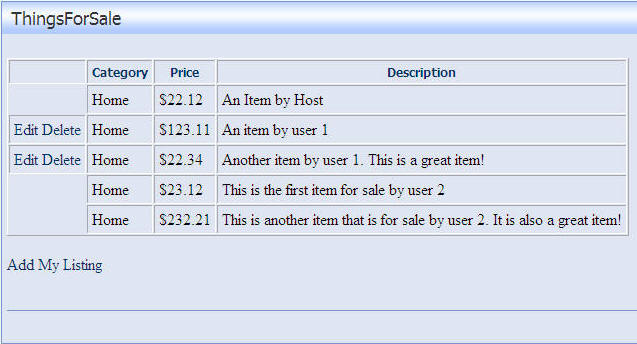
Ok sounds good, I started working on the module. This is the code I have for my data access layer:
Public Class ThingsForSaleController
Public Shared Sub ThingsForSale_Insert(ByVal ThingsForSaleInfo As ThingsForSaleInfo)
DataProvider.Instance().ExecuteNonQuery("ThingsForSale_Insert", ThingsForSaleInfo.ModuleId, GetNull(ThingsForSaleInfo.UserID), GetNull(ThingsForSaleInfo.Category.ToString), GetNull(ThingsForSaleInfo.Description.ToString), GetNull(ThingsForSaleInfo.Price))
End Sub
Public Shared Sub ThingsForSale_Delete(ByVal ThingsForSaleInfo As ThingsForSaleInfo)
DataProvider.Instance().ExecuteNonQuery("ThingsForSale_Delete", ThingsForSaleInfo.ID)
End Sub
Public Shared Sub ThingsForSale_Update(ByVal ThingsForSaleInfo As ThingsForSaleInfo)
DataProvider.Instance().ExecuteNonQuery("ThingsForSale_Update", ThingsForSaleInfo.ID, ThingsForSaleInfo.ModuleId, GetNull(ThingsForSaleInfo.UserID), GetNull(ThingsForSaleInfo.Category.ToString), GetNull(ThingsForSaleInfo.Description.ToString), GetNull(ThingsForSaleInfo.Price))
End Sub
Public Shared Function ThingsForSale_SelectAll(ByVal ModuleId As Integer) As List(Of ThingsForSaleInfo)
Return CBO.FillCollection(Of ThingsForSaleInfo)(CType(DataProvider.Instance().ExecuteReader("ThingsForSale_SelectAll", ModuleId), IDataReader))
End Function
Private Shared Function GetNull(ByVal Field As Object) As Object
Return Null.GetNull(Field, DBNull.Value)
End Function
End Class
There is a simple class called “ThingsForSaleInfo” that I don’t show but trust me it is just a simple class to hold the properties of: ID, ModuleId, UserID, Category, Description, and Price.
The rest of my code is in the one web control. Most of the code I have so far is a GridView control to display the things for sale and a FormView control to allow a person to enter an item. These controls are directly bound to the Object Data Source control that is directly bound to the code above.
This is a massive reduction of code. At the same time is still adheres to the proper n-tier design. I am a programmer from back in the Cold Fusion 1.0 days and this is the fastest, yet at the same time, best structured way to program a web page that was ever created. The coming changes to the Data Access Layer to complement these changes is very exciting.
The thing that is frustrating though is that while the Generic methods will exists in the DotNetNuke 3 version (and will still save you a lot of code) the Object Data Sources wont because they will only work with ASP.NET 2.0. To implement the same module in DotNetNuke 3 without using Object Data Sources will take almost twice as much code.
I predict that Object Data Sources will have a profound impact on the way we program in the future. Their time has come.