I have just completed Part 2 of my DotNetNuke Linq to SQL tutorial. Using the techniques covered in this tutorial I have been able to reduce my development time by at least 50%. In some cases where I had a lot of tables in my module, I have been able to reduce development time by 75%.
Along with this, I am also creating more efficient SQL. In the past I would create Data Access Layer methods that would select all fields and update all fields in a table each time they are called. With Linq to SQL only the fields that actually need to be selected or updated are used in the SQL statement. This has increased the performance of the application.
Linq to SQL also provides concurrency detection automatically. If one user alters a record after another user has pulled it up it will detect this and automatically prevent the update (you can handle the resolution of concurrency issues in a number of different ways).
The latest tutorial covers these real world situations:
Category Administration
An example of the code savings can be illustrated by the steps required to "Show the number of items in each category":
Double-click on Categories.ascx to open it. Switch to Design view.
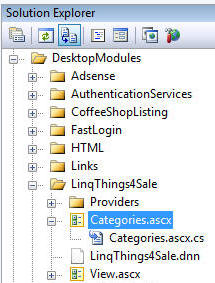
Select the LinqDataSource1 control. In it's Properties, click the yellow "lighting bolt" to view it's events. Double-click on the box to the right of Selecting.
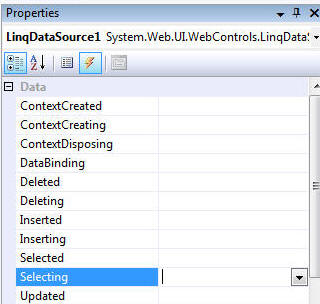
This will wire-up a method. Alter the method so it reads:
protected void LinqDataSource1_Selecting(object sender, LinqDataSourceSelectEventArgs e)
{
LinqThings4SaleDataContext LinqThings4SaleDataContext = new LinqThings4SaleDataContext();
var Things4Sale = from T in LinqThings4SaleDataContext.ThingsForSale_Categories
select new
{
T.ID,
T.Category,
Count = LinqThings4SaleDataContext.ThingsForSales.Count(c => c.Category == T.Category)
};
e.Result = Things4Sale;
}
(note: normally you would place data access code in a separate file. This code was placed inline only for brevity)
Click on the options for the GridView and select Edit Columns...
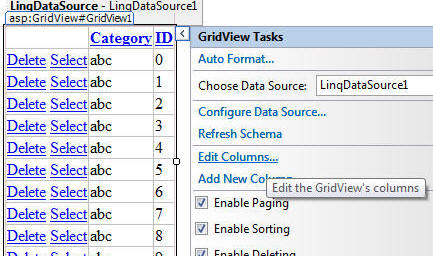
Click the Add button.
An extra ID and Category field will be added to the Selected fields box. Use the X button to remove the extra ID field. Click on the extra Category field and in the BoundField properties box:
- Change HeaderText to Count
- Change SortExpression to Count
- Change DataField to Count
Click the OK button.
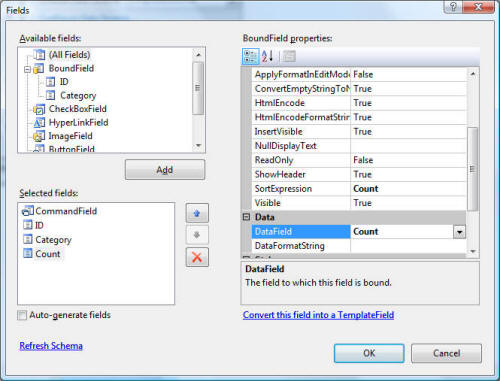
When you view the Category administration in the website you will be able to see the count of items using that Category and still sort and page the GridView.
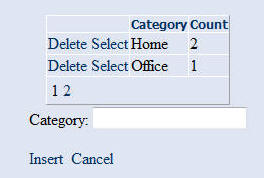
The tutorial can be found here:
Creating a DotNetNuke® Module using LINQ to SQL For absolute beginners! Part 2