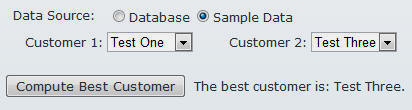
At my day job we have an advanced developer who was given a difficult project where he has to implement a dozen business rules. I’m talking “ask for a blessing before you go in” and “ask for forgiveness when you come out” complicated code.
I watched him work on the code, and he is methodical at writing down the rules and writing a test case to make sure he properly implemented it. This usually involves creating a “test case” by setting up data in the database just right and then clicking buttons and links and checking the expected output. I have worked with him over a year, and I can assure you he has probably deleted more good code than I have ever written. Everything is properly structured and segmented and the overall design will bring tears to you eyes with the beauty of it’s implementation.
But management keeps asking for major changes.
So I see this developer re-think his design and carefully re-factor his code to accommodate huge changes in requirements. He carefully retests all the existing business rules as he implements the new ones. Sometimes it takes hours for him to properly test everything. The guy is a total pro and he gets the job done.
But, here is the problem. He is a contract programmer, and when he moves on to his next job guess who has to maintain the code… me!
I had a nightmare where management came up to me and they wanted to “enhance” that code “one more time”. How can I make management understand that it could take me 6 hours to make just one small change to the code? Yes the code is well designed but it simply cannot anticipate all the requirements that are being demanded of it. It constantly has to be re-factored and each re-factoring brings a very real risk of breaking existing code.
Unit Testing
I am sure we have all read blogs on “getting started with unit testing”. They show a simple test that calls a method that returns some prime number. First the test fails because the code has not been written, next, they write the code and the test passes. “Wow this is great” you say, and then look for some examples where they connect to the database.
Now you are wading through a lot of complicated examples to Initialize() and Cleanup() to get the data just right. You are actually spending considerable time to “set-up a valid test” and then return the data to the previous state so the test is repeatable. When you realize that there is more code to “properly Unit Test” than there is in the project it’s self you come to the conclusion that it is not worth it. Just fix the bugs as they come, it will take less time.
However, in this case I needed Unit Tests to manage the complexity of the business rules. I really don’t care about “proper code coverage” and “proper isolation”, I just want something to help me so I don’t lie awake at 2:00 am thinking about accidently breaking some very important code.
Easy Unit Business Rule Testing
There is wonderful stuff out there on Unit Testing but I needed something simple and easy. A Unit Test purist would pass out from shock at the manner I butcher the normal Unit Testing paradigm.
The main thing I did was only test the specific methods I cared about, and only for business rule adherence. I load up a bunch of sample data and then toss it into a business object and check the expected result. Since I am using Linq to SQL, it is easy to implement the ‘Repository Pattern” and substitute my sample tables for real ones. The code doesn’t realize that it is not connecting to a real database and produces the same output for the same input every time.
But understand, when others talk about Unit testing, this is not what they mean. You should be testing each method inside the business object. If all tests pass you then know that the any re-factoring “should” not have broken anything.
This is Unit Testing for the guy who buys Life Insurance only if he plans to travel. I just call the methods on the “outside” of the business object and ask for an expected result. If I need to, I can test specific methods “on the inside” (methods that are called by the primary methods).
So it isn’t really Unit Testing, I guess it’s “Business Rule Testing”.
You can read the article here:
http://www.adefwebserver.com/DotNetNukeHELP/Misc/UnitTesting/