On Monday, we launched the Razor DotNetNuke Hackathon with a meeting of SEADUG (Seattle DotNetNuke User Group) held at Microsoft offices in beautiful downtown Bellevue.
For those of you proposing to write Razor “scripts” as part of the Hackathon, I have decided to write a series of short Tips and Tricks.
In this fourth post I will cover how you can go about extending the provided helpers to provide a simpler API for the scripts to consume.
The DotNetNukeWebPage class, which is the base class for all Razor scripts used in DotNetNuke has three additional properties.
Figure 1 – DotNetNukeWebPage Proeprties |
1: Protected Friend Property Dnn As DnnHelper
2: Protected Friend Property Html As HtmlHelper
3: Protected Friend Property Url As UrlHelper
|
In this tip we are going to extend the Dnn property to add an extra method. The Dnn property is an instance of the DnnHelper class which is defined in the DotNetNuke.Web.Razor library.
Figure 2 - The DnnHelper Class
|
1: Public Class DnnHelper
2:
3: Private _context As ModuleInstanceContext
4:
5: Public Sub New(ByVal context As ModuleInstanceContext)
6: _context = context
7: End Sub
8:
9: Public ReadOnly Property [Module] As ModuleInfo
10: Get
11: Return _context.Configuration
12: End Get
13: End Property
14:
15: Public ReadOnly Property Tab As TabInfo
16: Get
17: Return _context.PortalSettings.ActiveTab
18: End Get
19: End Property
20:
21: Public ReadOnly Property Portal As PortalSettings
22: Get
23: Return _context.PortalSettings
24: End Get
25: End Property
26:
27: Public ReadOnly Property User As UserInfo
28: Get
29: Return _context.PortalSettings.UserInfo
30: End Get
31: End Property
32:
33: End Class
|
Imagine that you want to write a script which lists all of the roles on the site. You could use the full DotNetNuke API in your Razor script as shown in Figure 3
Figure 3 – Role List script
|
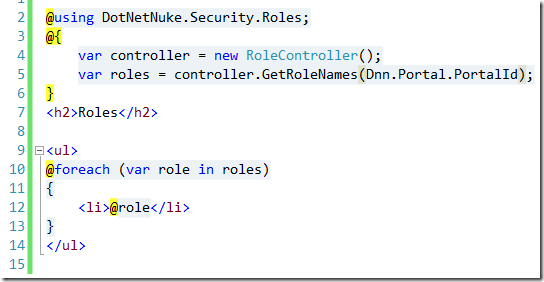 |
There is nothing wrong with this – it works. But what if you could have a much simpler script to do the same task (Figure 4).
Figure 4 – Simplified Role List script
|
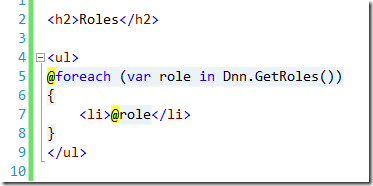 |
For beginner developers who are trying their hand at DotNetNuke development using the Razor Host module, this is much more intuitive. But GetRoles() is not a member of the DnnHelper class, and I don’t want to modify a core class. This is where the wonders of Extension methods comes in. Since C# 3 (or VB 9) which shipped with Visual Studio 2008, we have been able to add functionality to existing classes, without modifying the class directly.
In a separate project, I added a class DnnHelperExtensions, where I defined the following extension method (Figure 5).
Figure 5 – GetRoles Extension Method
|
1: public static class DnnHelperExtensions
2: {
3: public static List<String> GetRoles(this DnnHelper helper)
4: {
5: var controller = new RoleController();
6: var _roles = new List<String>(controller.GetRoleNames(
7: helper.Portal.PortalId
8: ));
9:
10: return _roles;
11: }
12: }
|
This method hides the DotNetNuke complexity from the scripter. We don’t need to worry about what namespace(s) to include in our script and we don’t care that GetRoleNames needs a PortalId as the helper method takes care of it.
There is one final step that we need to do to get this to work. We need to add a reference to the Helpers namespace in the web.config file that lives in the RazorModules folder.
Figure 6 – Updating web.config
|
1: <system.web.webPages.razor>
2: <pages pageBaseType="DotNetNuke.Web.Razor.DotNetNukeWebPage">
3: <namespaces>
4: <add namespace="Microsoft.Web.Helpers" />
5: <add namespace="WebMatrix.Data" />
6: <add namespace="DotNetNuke.Web.Razor.Helpers" />
7: </namespaces>
8: </pages>
9: </system.web.webPages.razor>
|
In the final release of the Razor Host Module, we will add the DotNetNuke.Web.Razor.Helpers namespace to the web.config included, and we will start to flesh out the DnnHelper class with some more intuitive methods.
However, if ever ever feel that something is missing you can create your own. Just make sure that all your helper Extension Methods are in the same namespace and all you will need to do is add the assembly to the bin folder, and they will just work.
Figure 7 – The Resulting Razor Script in Action.
|
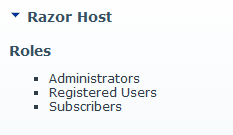 |
This post is cross-posted from my personal blog.