This post is cross-posted from my personal blog.
Last week, shaun posted a blog in which he discussed whether DotNetNuke (DNN) should be rewritten in ASP.NET MVC. This blog created quite a stir, both within the DotNetNuke Community and within the ASP.NET Community as a whole.
In a comment that I added to shaun’s post I suggested that the debate should not be over whether DNN should be rewritten in ASP.NET MVC (or any other framework that may come in the future), the discussion should be on “How can we enable developers to use the ASP.NET Technologies of their choice when developing extensions for DNN.” After all, if we rewrite DNN in MVC we effectively lock out WebForm developers.
Thanks to an idea from my son Andrew (much of what I used in this work was from his Maverick project on codeplex) I have been able the create a Framework that sits on top of DotNetNuke (and System.Web.MVC) and allows modules to be created using the ASP.NET MVC Framework (DotNetNuke.Web.Mvc).
In this article I will review how – using this add-on layer we can go about creating a simple ASP.NET MVC module. First, add an ASP.NET MVC Application to your Visual Studio solution. (See Figure 1 below)
Figure 1 – Add a new MVC Web Application in the Desktop Modules folder called MVC_Test
|
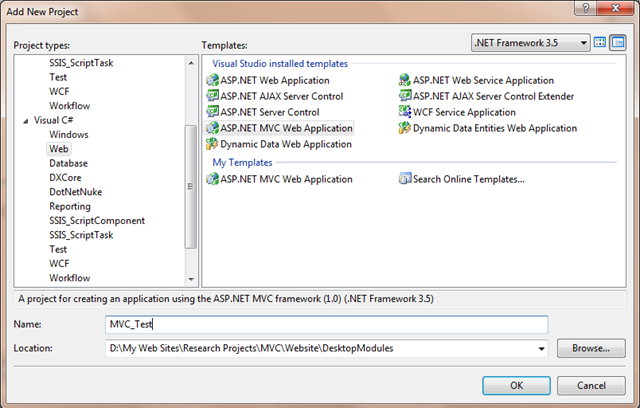 |
Note that the location of the project is in the DesktopModules folder of our test website. This is exactly the same process we would use to add a WAP (Web Application Project) style module. Figure 2 below shows the Solution Explorer after adding this project.
Figure 2 – The new Project in Solution Explorer
|
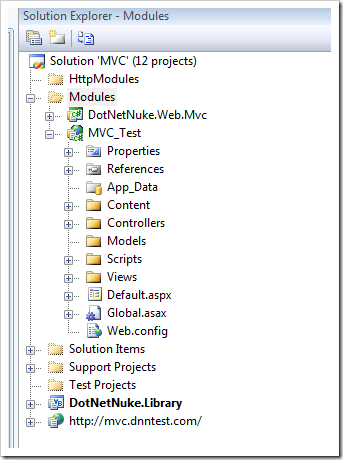 |
Before we go any further – we should prove to ourselves that this is a valid ASP.NET MVC Web Application, by selecting the Default.aspx file, and selecting View in Browser from the Context menu (see Figure 3 below)
Figure 3 – Browsing to the site demonstrates that this is a valid ASP.NET MVC Application
|
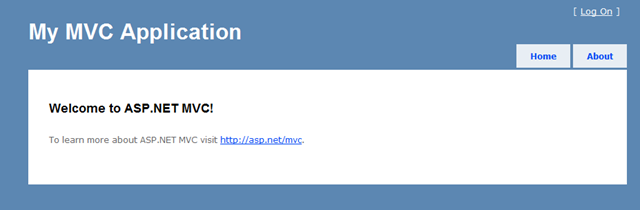 |
So now we have an MVC Application, how do we make it run as a Module in our DotNetNuke website? First we need to add a new class to our project – MVC_TestApplication.cs (see Figure 4).
Figure 4 – Add a new class MVC_TestApplication to the root of the Application
|
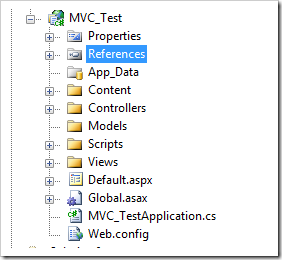 |
And we need to add references to our DotNetNuke Library project and to the new DotNetNuke.Web.Mvc project (see Figure 5)
Figure 5 – Add references to the DotNetNuke Library and the new DotNetNuke.Web.Mvc project
|
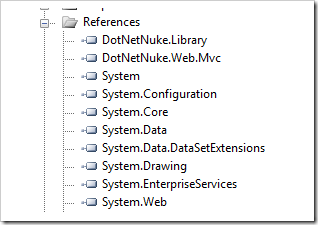 |
When we have done that we need to open the new class we added and add some very simple code.
Listing 1 – The MVC_TestApplication class
|
1: using System.Web.Routing;
2: using DotNetNuke.Web.Mvc;
3: using DotNetNuke.Web.Mvc.Routing;
4:
5: namespace MVC_Test
6: {
7: public class MVC_TestApplication : MvcModuleApplication
8: {
9: protected override string FolderPath
10: {
11: get { return "MVC_Test"; }
12: }
13:
14: protected override void Init()
15: {
16: base.Init();
17: RegisterRoutes(Routes);
18: }
19:
20: private static void RegisterRoutes(RouteCollection routes)
21: {
22: routes.RegisterDefaultRoute("MVC_Test.Controllers");
23: }
24: }
25: }
|
The most important thing to note is that this class inherits from MvcModuleApplication, a new base class in the new DotNetNuke.Web.Mvc project. The Init method allows us to register any routes that our MVC Module Application will need and the FolderPath property tells the DotNetNuke.Web.Mvc project where our Views are located. Finally we need to make a very small change to the default View (Listing 2).
Listing 2 – Index.aspx
|
1: <%@ Page Language="C#" MasterPageFile="../Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage" %>
2:
3: <asp:Content ID="indexTitle" ContentPlaceHolderID="TitleContent" runat="server">
4: Home Page
5: asp:Content>
6:
7: <asp:Content ID="indexContent" ContentPlaceHolderID="MainContent" runat="server">
8: <h2><%= Html.Encode(ViewData["Message"]) %>h2>
9: <p>
10: To learn more about ASP.NET MVC visit <a href=http://asp.net/mvc
title="ASP.NET MVC Website">http://asp.net/mvca>.
11: p>
12: asp:Content>
|
If you blink you won’t see the change.
The change is to the reference to the MasterPageFile – the value of the attribute is changed from “~/Views/Shared/Site.Master” to “../Views/Shared/Site.Master”. The reference points to the same file – but the original reference assumes that the Views folder is at the root of the IIS Application – as a module it is actually at ~/DesktopModules/MVC_Test/Views”. By making the reference relative it will work in both scenarios.
Next we need to create our Module Extension. This is done in much the same way as we do today, the only difference being that when we register the Module Control we use the Namespace for the new class that we added – MVC_Test.MVC_TestApplication (See Figure 6 below). Since version 5.0, DNN has allowed module controls to be server controls and ultimately our new MVC_TestApplication class inherits from Control.
Figure 6 – Add the MVC_TestApplication as the Source for the Default Module Control
|
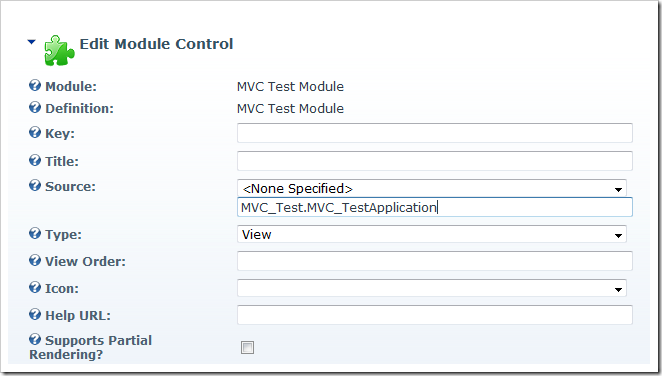 |
Finally build our new MV Module Application and copy the assembly from the bin folder of the project to the bin folder of the Website.
We are now ready to see if everything works. Add a new Page and add the newly registered Module to the Page and “hey presto” we get a Module that looks like the MVC Application (See Figure 7 below)
Figure 7 – Add the Module to the Page
|
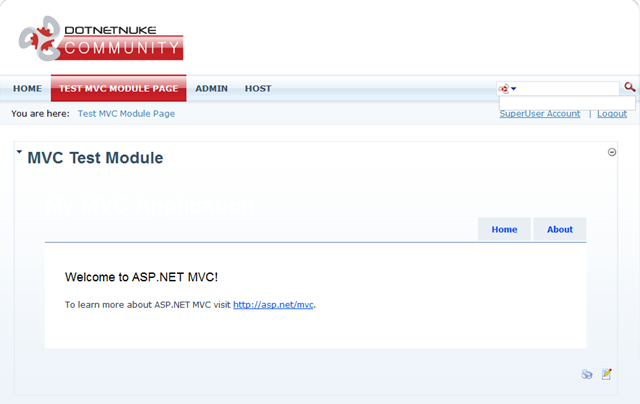 |
The really cool thing is that we can still browse to the site (as an MVC Application) by selecting the Default.aspx page, and selecting View in Browser from the context menu, as we did in Figure 3 above.
Conclusions
In summary I have demonstrated in this article, that using ASP.NET MVC for Module Development is a possibility. There still are a number of issues to resolve however, including, but not limited to:
- Handling routes other than the default route.
- Do the Html Helpers in ASP.NET MVC still work? and if not how can we make them work?
- As Webforms allows only one Form tag which is defined in the base page -Default.aspx -how do we handle Forms in an MVC Module.
- Issues around the use of Master pages and styles, and DNN skinning – we can solve this by removing the dependency on Master pages which is not a requirement of ASP.NET MVC.
I don’t expect any of these to be show-stoppers, but much more work still needs to be done. In future blogs, I will describe how what I describe in this article was done and report on my attempts to resolve the remaining issues.